How to Read Data from Realtime Database ?
Follow the steps to read data from Firebase Realtime Database for Web :
- Setup Firebase SDK and Initialize Realtime Database.
There are 2 method to read data,
Using get( ) :
get return a 'Promise' so to handle it we need to make 'async function'.
import { database } from '<YOUR_FIREBASE_CONFIG_FILE_PATH>';
import { ref, get } from 'firebase/database';
async function readData() {
const snapshot = await get(ref(database, 'REFERENCE_PATH'));
const data = await snapshot.val();
// data containe the value that is Read from Database
}
readData();
get(ref(database, 'REFERENCE_PATH'))
.then((snapshot) => {
//'snapshot.exists' checks data is available or its null
// And return boolean value
if (snapshot.exists) {
data = snapshot.val();
}
})
.catch((error) => console.log(error));
2. Using onValue( )
It make a connection to database and calls automatically every time when data is changed at
REFERENCE_PATH
and get updated value. (It helps when you are making app like chat app.)import { database } from '<YOUR_FIREBASE_CONFIG_FILE_PATH>';
import { onValue, ref } from 'firebase/database';
onValue(ref(database, 'REFERENCE_PATH'), (snapshot) => (data = snapshot.val()));
You can also get data only once by adding object like this,
import { database } from '<YOUR_FIREBASE_CONFIG_FILE_PATH>';
import { onValue, ref } from 'firebase/database';
onValue(
ref(database, 'REFERENCE_PATH'),
(snapshot) => (data = snapshot.val()),
{ onlyOnce: true }
);
snapshot.val()
using 'useState' hook or make 'let' variable to get updated data.let data;
Use this line above onValue() and get updated values in
data
variable.Related Post
Write data
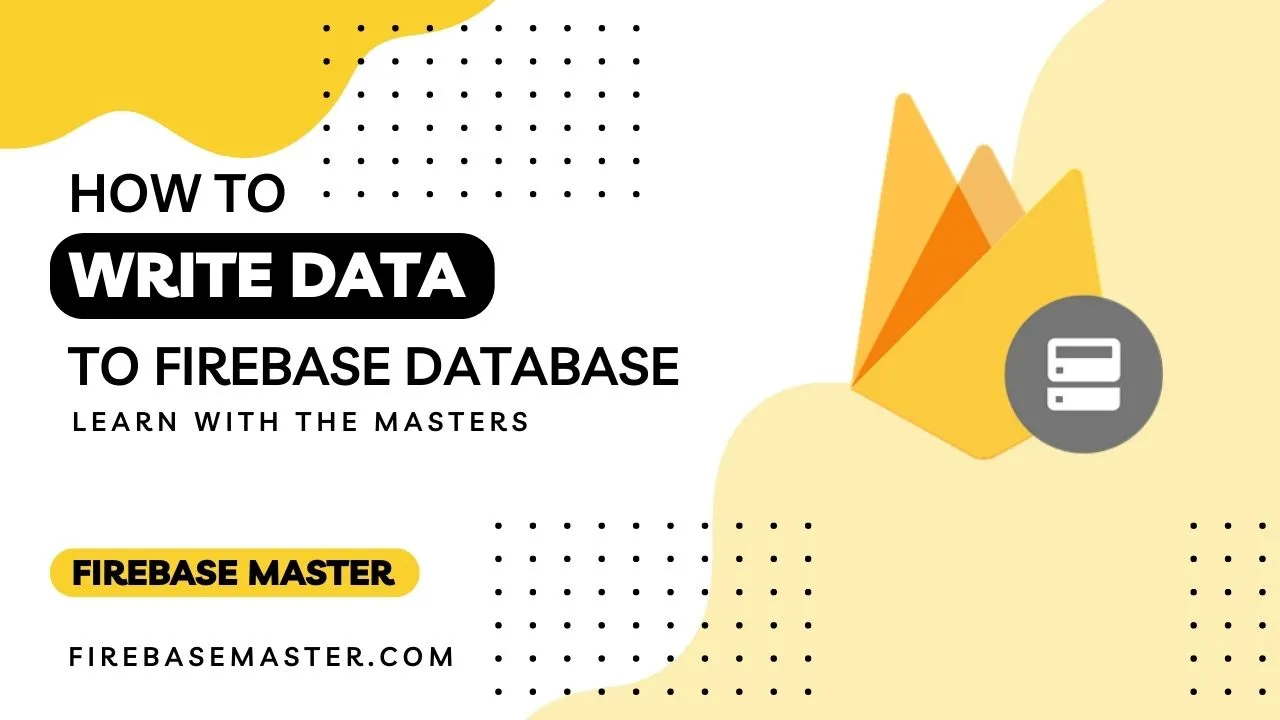
Update and Delete data
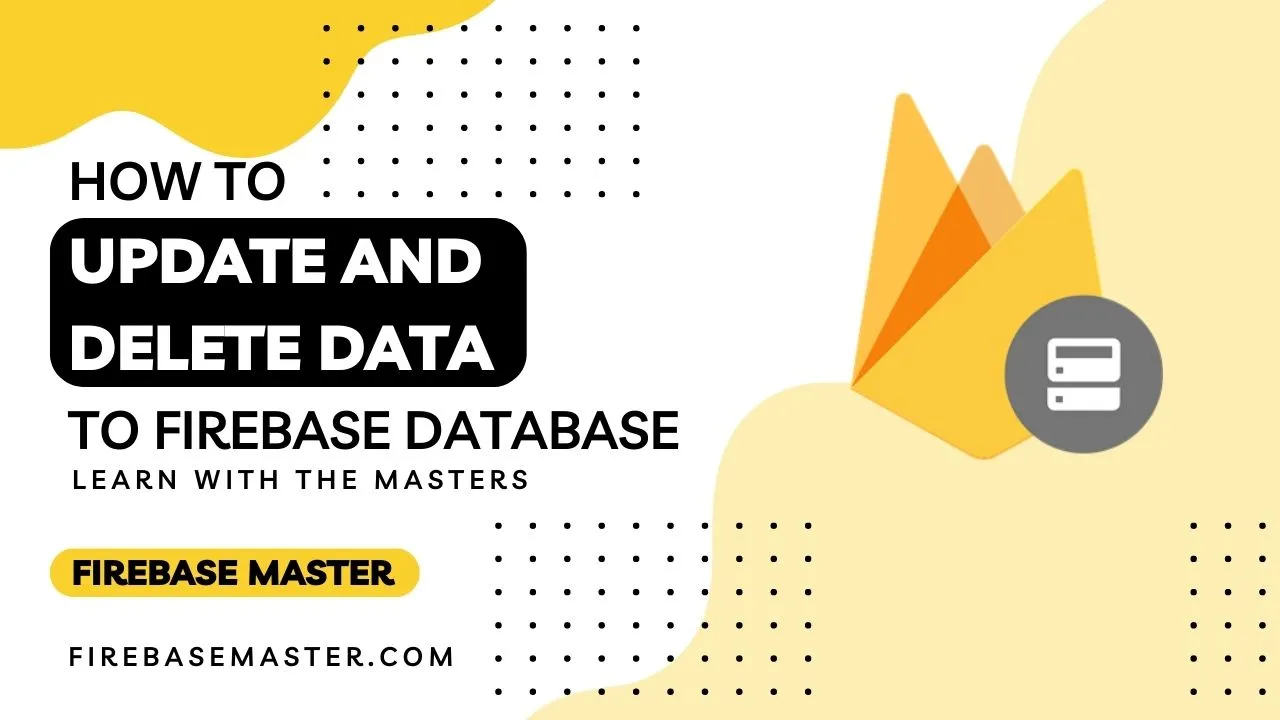
Setup and Initialize
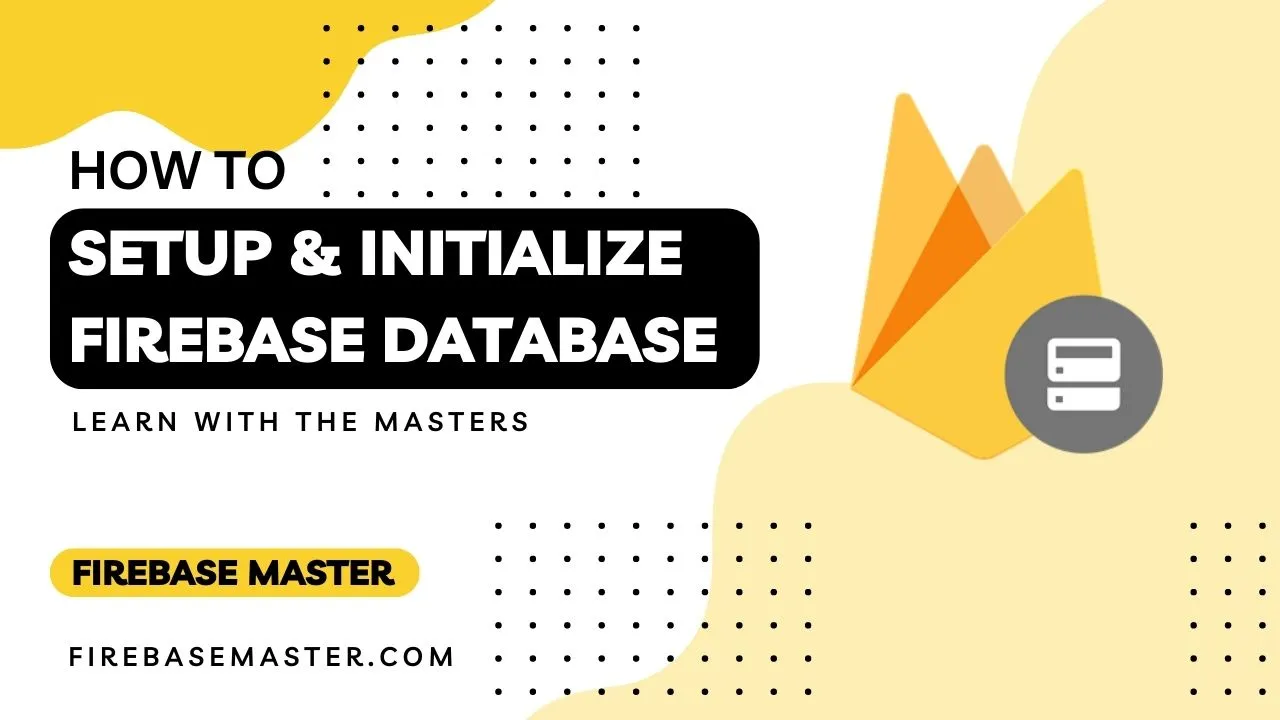
Explore
Copyright © 2024, All rights reserved by Firebase Master