How to Upload File to Firebase Storage ?
Follow the steps to Upload File to Firebase Storage for Web :
- Setup firebase SDK and initialize storage then export storage so you can use it in other files.
- Now import storage reference from 'firebaseConfig' file.
Upload Files :
import { storage } from '<YOUR_FIREBASE_CONFIG_FILE_PATH>';
import { ref, uploadBytes } from 'firebase/storage';
uploadBytes(ref(storage, 'REFERENCE_PATH'), 'FILE');
Set REFERENCE_PATH
as location of file along with file name where you want to save in storage bucket.
Pass FILE
that you want to upload on storage by taking input from user or any other way.
For example,
Upload an image to firebase storage named 'firebasemaster.png' in 'album' folder by taking input from user using input element.<label for="file">Choose file to upload</label>
<input type="file" id="file" name="file">
This way, you can get that
FILE
,const FILE = document.getElementById('file').files[0]
Now you can upload this FILE to firebase storage.
import { storage } from '<YOUR_FIREBASE_CONFIG_FILE_PATH>';
import { ref, uploadBytes } from 'firebase/storage';
uploadBytes(ref(storage, 'album/firebasemaster.png'), FILE);
File is uploaded to firebase storage in album as you can see in this image.
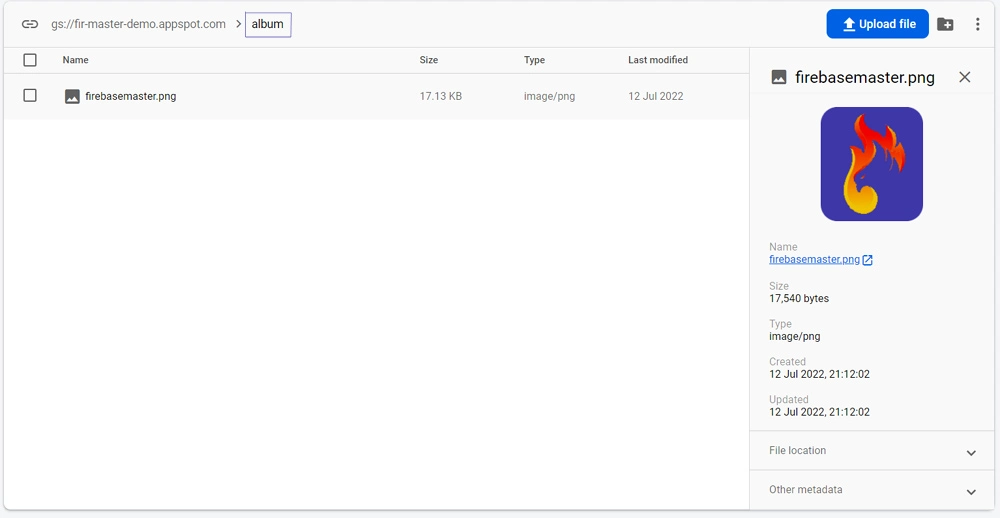
This return 'Promise' so it can be handled by using '.then()' or 'async/await'.
You can run any function or particular code after uploaded and also handle error control.
- .then( ) :
uploadBytes(ref(storage, 'album/firebasemaster.png'), FILE)
.then((snapshot) => {
// Run code after successful uploading
})
.catch((error) => console.log(error));
- async/await :
async function uploadFile() {
try {
const snapshot = await uploadBytes(
ref(storage, 'album/firebasemaster.png'),
FILE
);
} catch (error) {
console.log(error);
}
}
uploadFile();
Related Post
Setup and Initialize
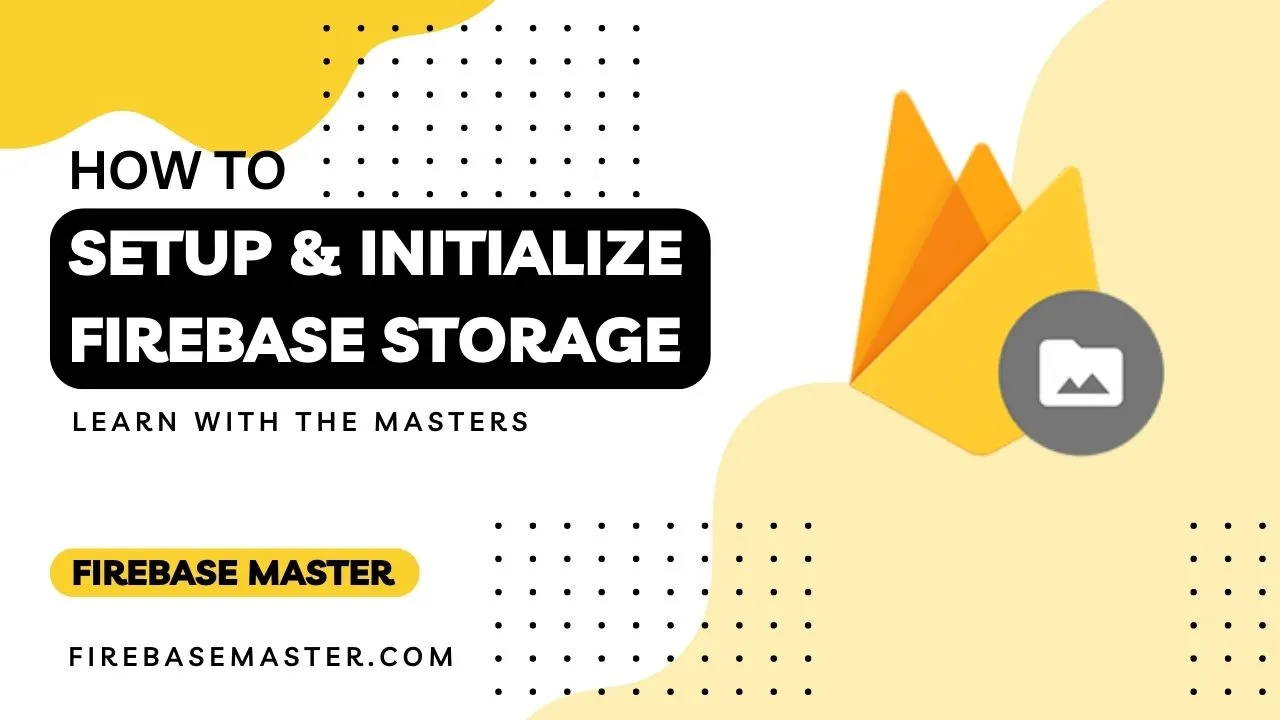
Explore
Copyright © 2024, All rights reserved by Firebase Master