Authenticate User Using Email Link (Passwordless)
You have one way to authenticate user using Email and Password(that is set by user) and also have other way to Sign-Up and Log-In user using Email Link(Passwordless) method.
In this method, user receive an mail that contain a authentication link. User is verified and redirected to your site with an token by clicking on that link. Token is presented as query parameter in the URL. User is authenticated and able to Sign-up and Log-in without any password by using token that is provided by Firebase's Email Link Authentication method.
Follow the steps to implement Passwordless authentication method.
Step 1. Enable Email Link sign-in method
- Open your project console and navigate to
Build
>Authentication
dashboard in that open Sign-in method tab. - Click on
Email/Password
that is in Native Provider column. - Now enable Email/Password and Email link(Passwordless) provider and hit Save button.
- If you haven't setup firebase SDK and initialize authentication then do it first.
Step 2. Send an authentication link to the user's email address
url
:Provide URL address where user is redirected back by clicking email authentication link with token. Domain name must be added in authorized to firebase console. If you are using custom domain then need to add that domain to Authorised Domains list by following this thatAuthentication
>Setting tab
>Authorised domains
and hit theAdd Domain
button.handleCodeInApp
:Set to true. It is expected that the user will be signed in and that the app will remember their Auth status.
Step 3. Sign-In user
url
with authentication token. Using that token user is able to sign in. You need to call some functions on that page where you redirected user to verify that token and log-in the user.Log-out User :
- signOut(auth) :
Related Post
Setup and Initialize
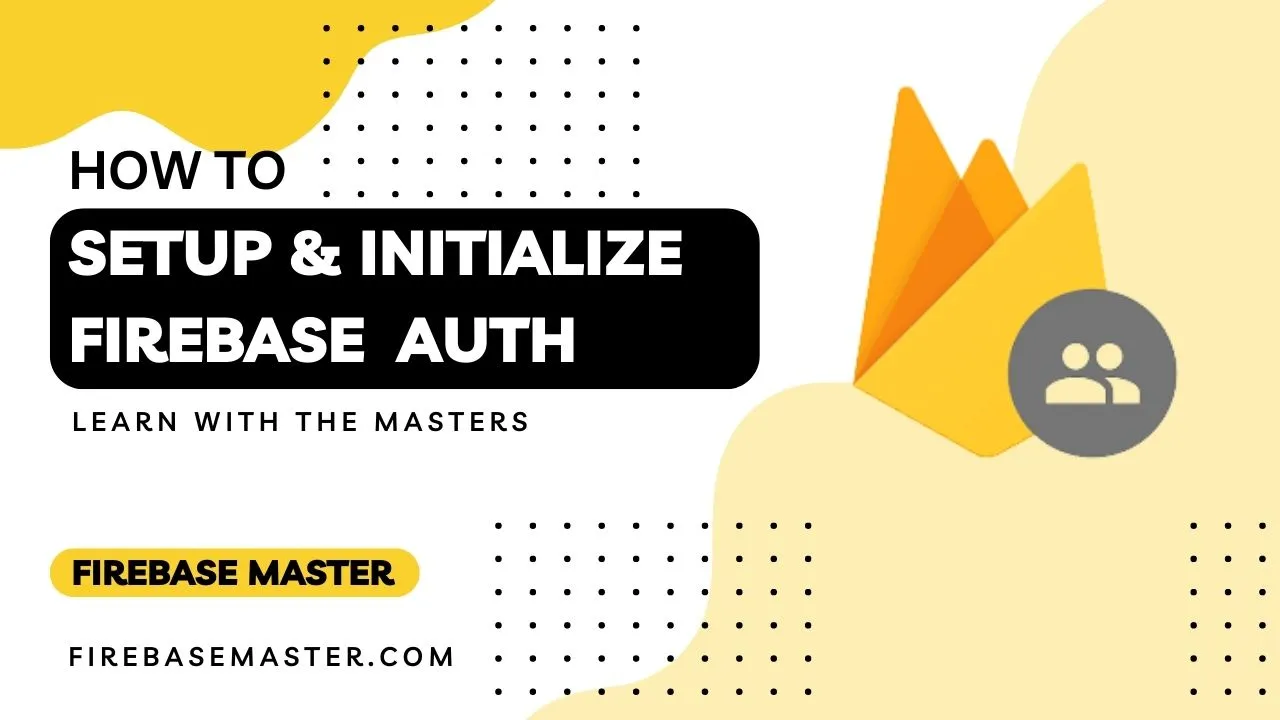
Phone number
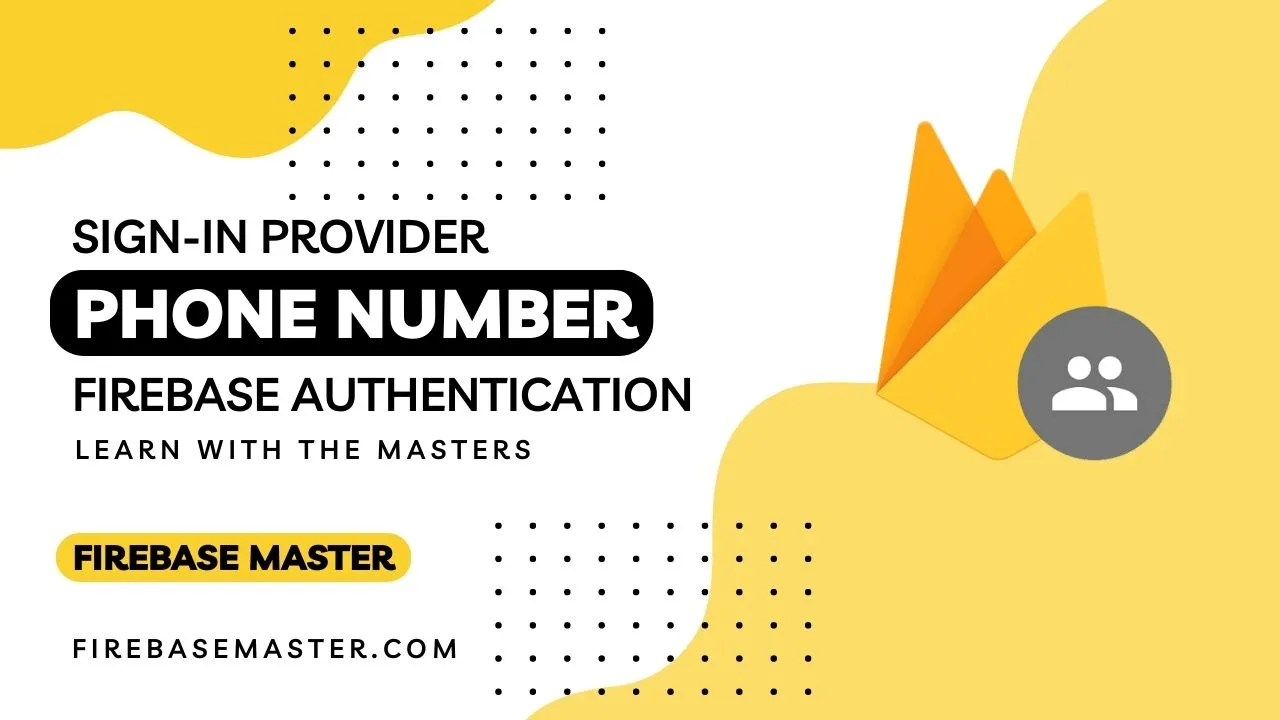
Email link (Passwordless)
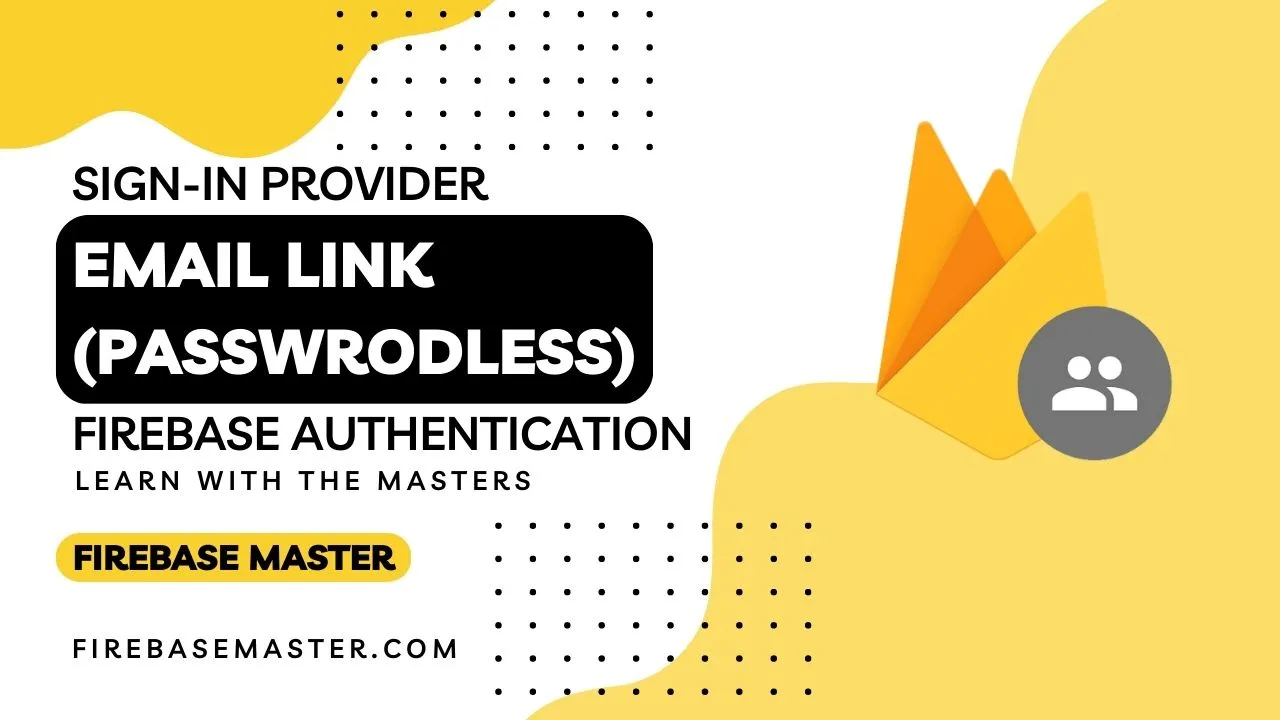
Google OAuth
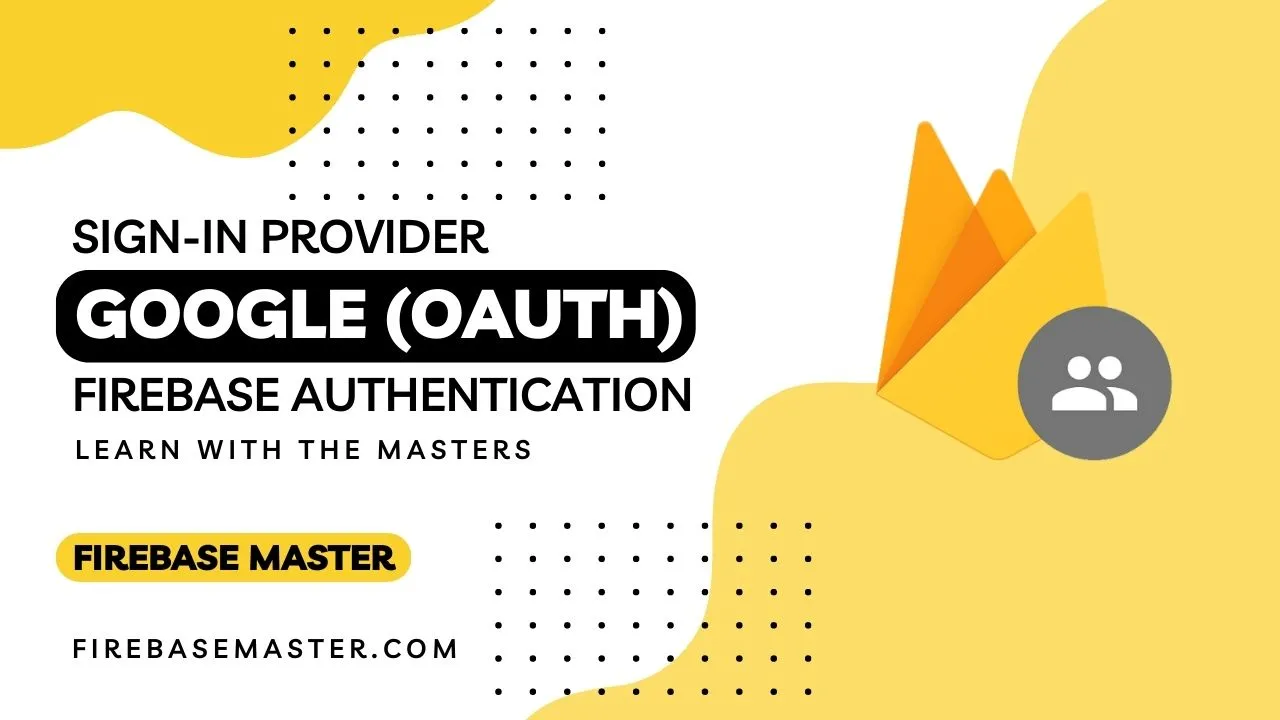
Explore
Copyright © 2024, All rights reserved by Firebase Master