Sign-In User using Google OAuth with Firebase
Using Google OAuth method provided by firebase, you can log-in user to your web app by user's google account and google OAuth provides an access token to you and also get user's display name, phone number, email id as well as uid (unique identity number) for your web app.
Step 1. Enable Google sign-in method for your project
- Open your project console and navigate to
Build
>Authentication
dashboard in that open Sign-in method tab and click on 'add new provider'. - Click on
Google
that is in Additional Provider column. - Now enable
Google
provider and hit Save button. - If you haven't setup firebase SDK and initialize authentication then do it first.
Step 2. Set up Sign-in flow
You just need to implement given code to Sign in user with Google OAuth. Simply call the
signInWithGoogle()
on the click of Sign-in button that you created.import { auth } from "<YOUR_FIREBASE_CONFIG_FILE_PATH>"; //see setup and initialize authentication if you have any doubt
import { signInWithPopup, GoogleAuthProvider } from "firebase/auth";
const signInWithGoogle = () => {
const provider = new GoogleAuthProvider();
auth.useDeviceLanguage(); // set browser language
signInWithPopup(auth, provider)
.then((result) => {
// write code to perform intended task
// like redirect user somewhere else after sign-in
// ...
// ...
// The signed-in user info.
const user = result.user;
console.log(user); // Provides user data as Object.
console.log(user.displayName); // User's display name.
console.log(user.email); // User's. Email ID.
console.log(user.phoneNumber); // User's phone number
console.log(user.photoURL); // User's photo URL
// ...
// This gives you a Google Access Token. You can use it to access the Google API.
const credential = GoogleAuthProvider.credentialFromResult(result);
const token = credential.accessToken;
// ...
})
.catch((error) => {
// Handle Errors here.
const errorCode = error.code;
const errorMessage = error.message;
// The email of the user's account used.
const email = error.customData.email;
// The AuthCredential type that was used.
const credential = GoogleAuthProvider.credentialFromError(error);
console.log(error);
// ...
});
};
You can use
signInWithPopup()
method to open a pop up window Of Google OAuth and help to sign in user to your web app by providing an access token.Log-out User :
- signOut(auth) :
import { auth } from '<YOUR_FIREBASE_CONFIG_FILE_PATH>';
import { signOut } from 'firebase/auth';
signOut(auth);
This method also return 'Promise' so you run functionality like redirecting user to log-in page after log-out using '.then()' or 'async/await'.
signOut(auth)
.then(() => {
// code for redirect user to Log-in page
// ...
})
.catch((error) => {
console.log(error);
});
Related Post
Google OAuth
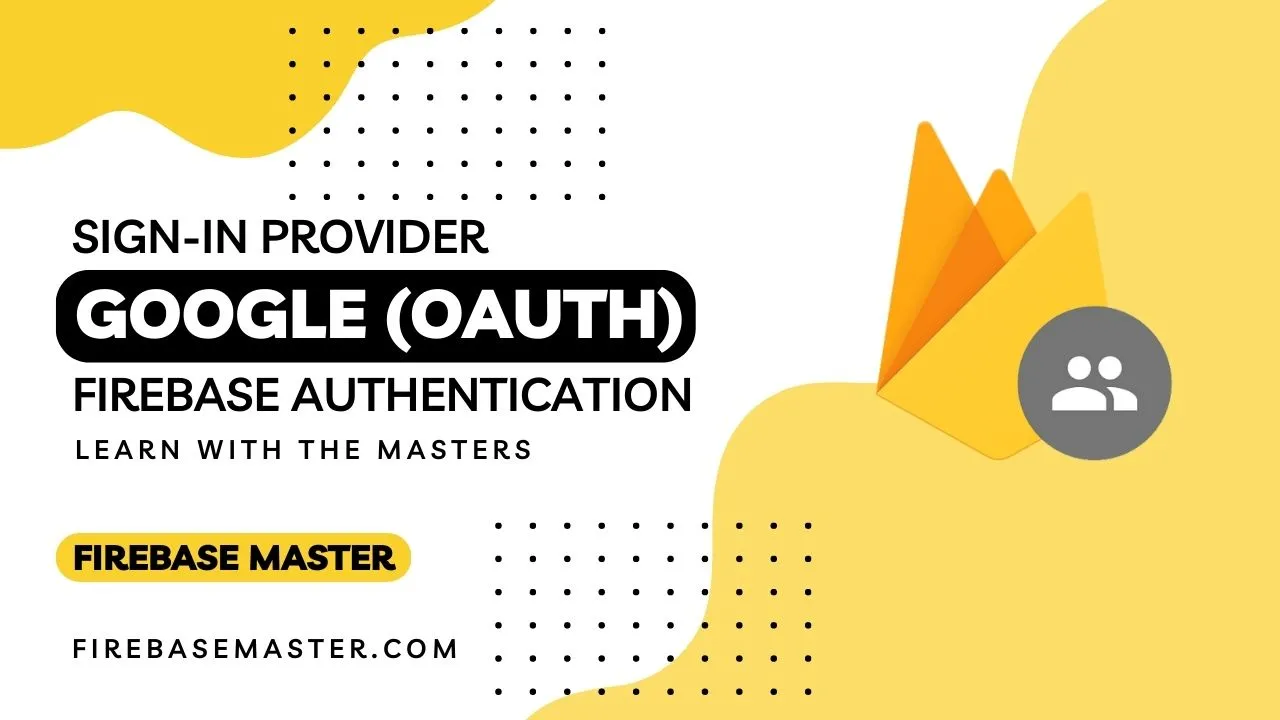
Email link (Passwordless)
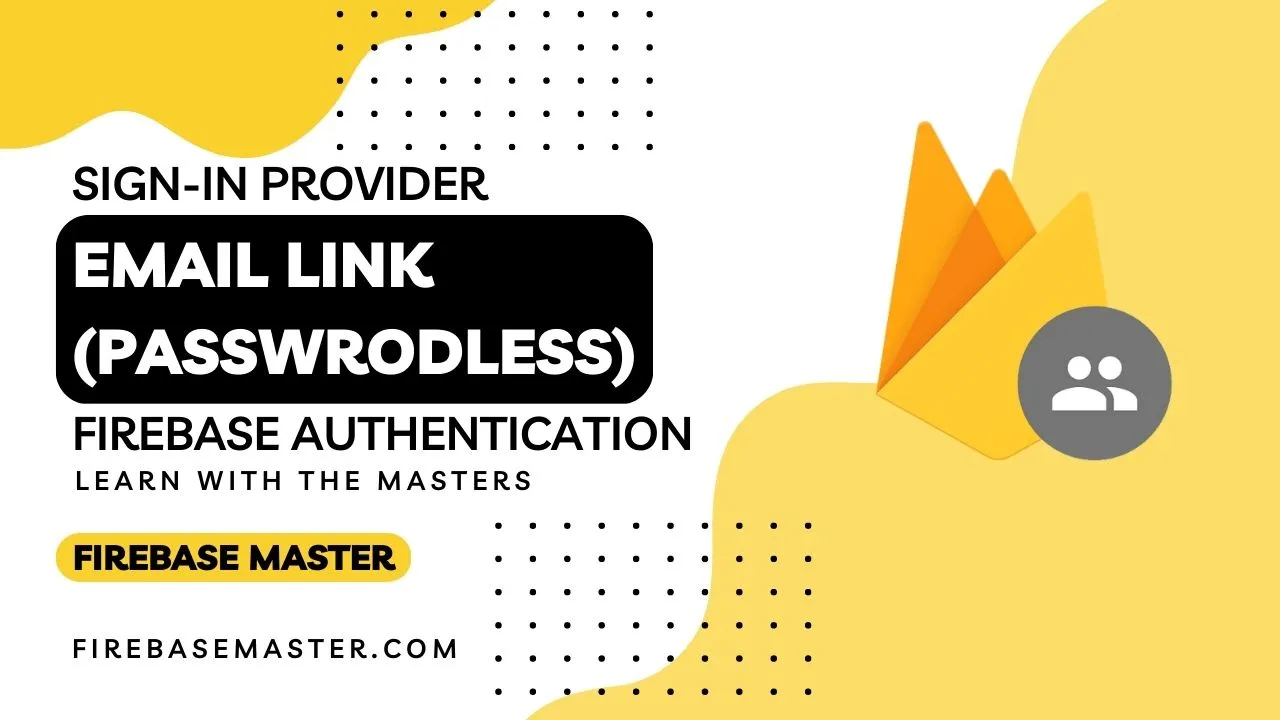
Email/Password
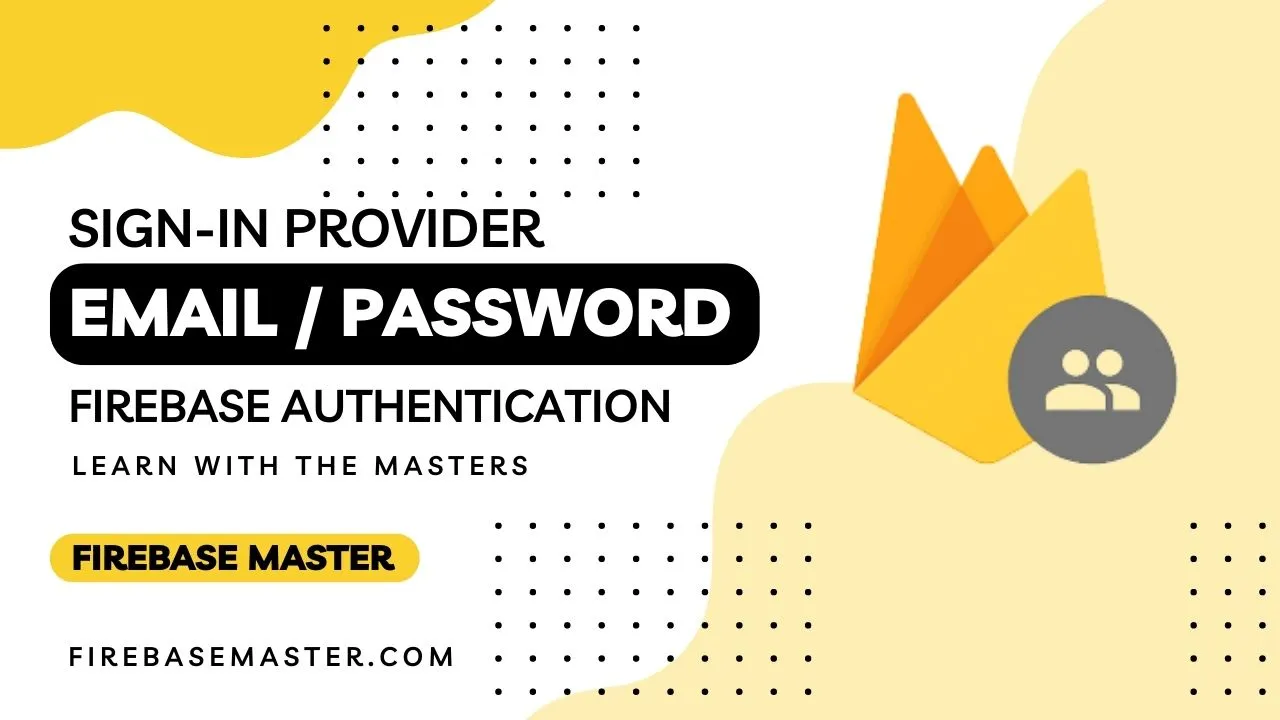
Setup and Initialize
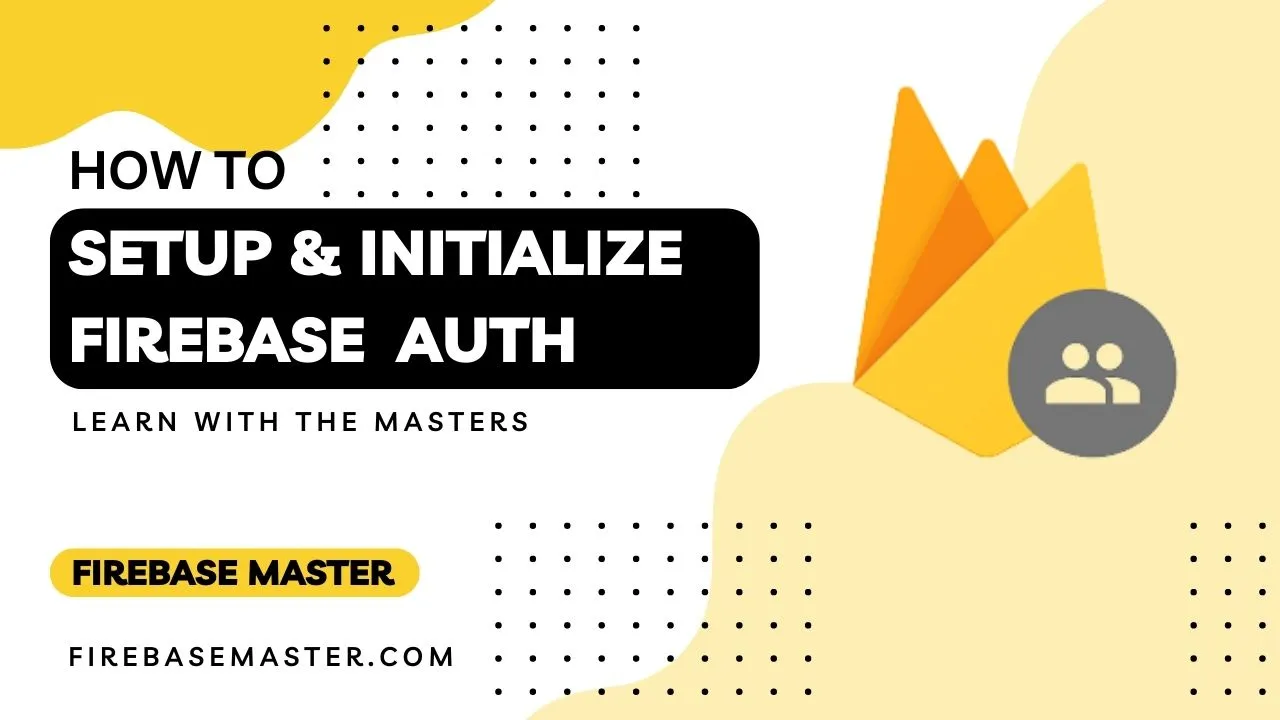
Explore
Copyright © 2024, All rights reserved by Firebase Master