How to Signup/ Login/ Logout User In Firebase Authentication ?
First enable "Email/Password" provider :
- Open your project console and navigate to
Build
>Authentication
dashboard in that open Sign-in method tab. - Click on
Email/Password
that is in Native Provider column. - Now enable Email/Password provider and hit Save button.
- After Clicking Save :
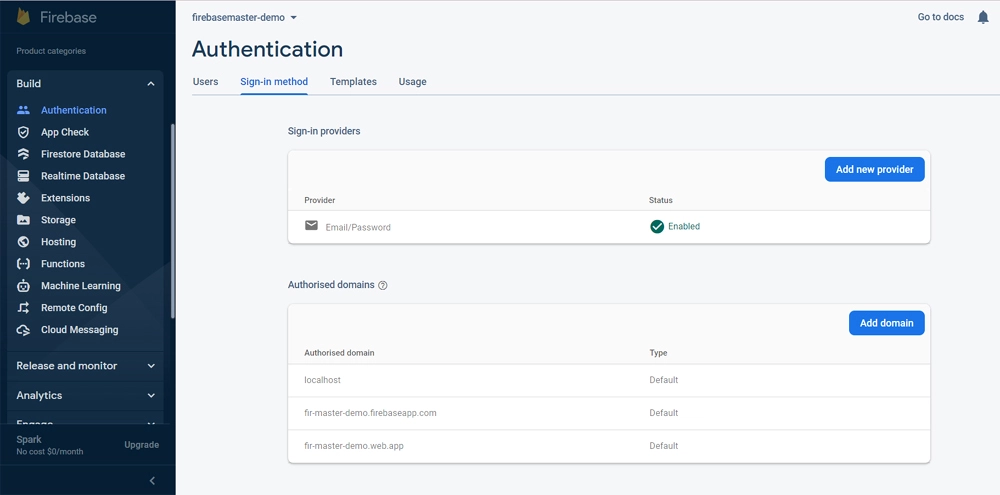
Create New User Account (Sign-up) :
- createUserWithEmailAndPassword(auth, 'EMAIL', 'PASSWORD') :
import { auth } from '<YOUR_FIREBASE_CONFIG_FILE_PATH>';
import { createUserWithEmailAndPassword } from 'firebase/auth';
createUserWithEmailAndPassword(auth, 'EMAIL', 'PASSWORD');
- EMAIL : Get Email address that is inputted by user and pass it in EMAIL or you can pass it manually by typing email in the method.
- PASSWORD : Get Password that is inputted by user and it in PASSWORD or you can pass it manually by typing password in the method.
For example,
You want to create new user and that's EMAIL
is 'you@firebasemaster.com' and PASSWORD
is '123456789'.
import { auth } from '<YOUR_FIREBASE_CONFIG_FILE_PATH>';
import { createUserWithEmailAndPassword } from 'firebase/auth';
createUserWithEmailAndPassword(auth, 'you@firebasemaster.com', '123456789');
After creating user account, you can see it in 'Users' tab in Authentication dashboard.
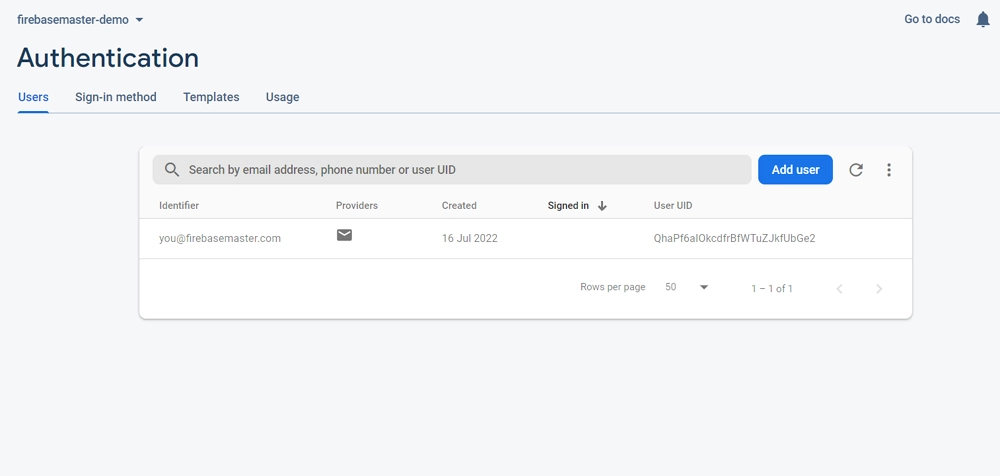
User will automatically login for first time after creating new user account. Firebase provides unique UID key to every user. You can see users email id but you can't see their password.
You can also add user through Authentication dashboard.
This method return 'user credentials' with 'Promise' so can be handled by using '.then()' or 'async/await' and get user credentials for app.
- .then( ) :
createUserWithEmailAndPassword(auth, 'EMAIL', 'PASSWORD')
.then((UserCredential) => {
const user = UserCredential.user;
})
.catch((error) => {
console.log(error.message);
});
- async/await :
async function createNewUserAccount() {
try {
const userCredential = await createUserWithEmailAndPassword(
auth,
'EMAIL',
'PASSWORD'
);
const user = userCredential.user;
} catch (error) {
console(error.message);
}
}
createNewUserAccount();
Log-in Existing User :
- signInWithEmailAndPassword(auth, 'EMAIL', 'PASSWORD') :
import { auth } from '<YOUR_FIREBASE_CONFIG_FILE_PATH>';
import { signInWithEmailAndPassword } from 'firebase/auth';
signInWithEmailAndPassword(auth, 'EMAIL', 'PASSWORD');
- EMAIL & PASSWORD : Get Email address & password that is inputted by user and pass it in EMAIL & PASSWORD or you can pass it manually by typing it in the method.
It is also return Promise so it can be handled by '.then()' or 'async/await'.
Log-out User :
- signOut(auth) :
import { auth } from '<YOUR_FIREBASE_CONFIG_FILE_PATH>';
import { signOut } from 'firebase/auth';
signOut(auth);
This method also return 'Promise' so you run functionality like redirecting user to log-in page after log-out using '.then()' or 'async/await'.
signOut(auth)
.then(() => {
// code for redirect user to Log-in page
// ...
})
.catch((error) => {
console.log(error);
});
Related Post
Setup and Initialize
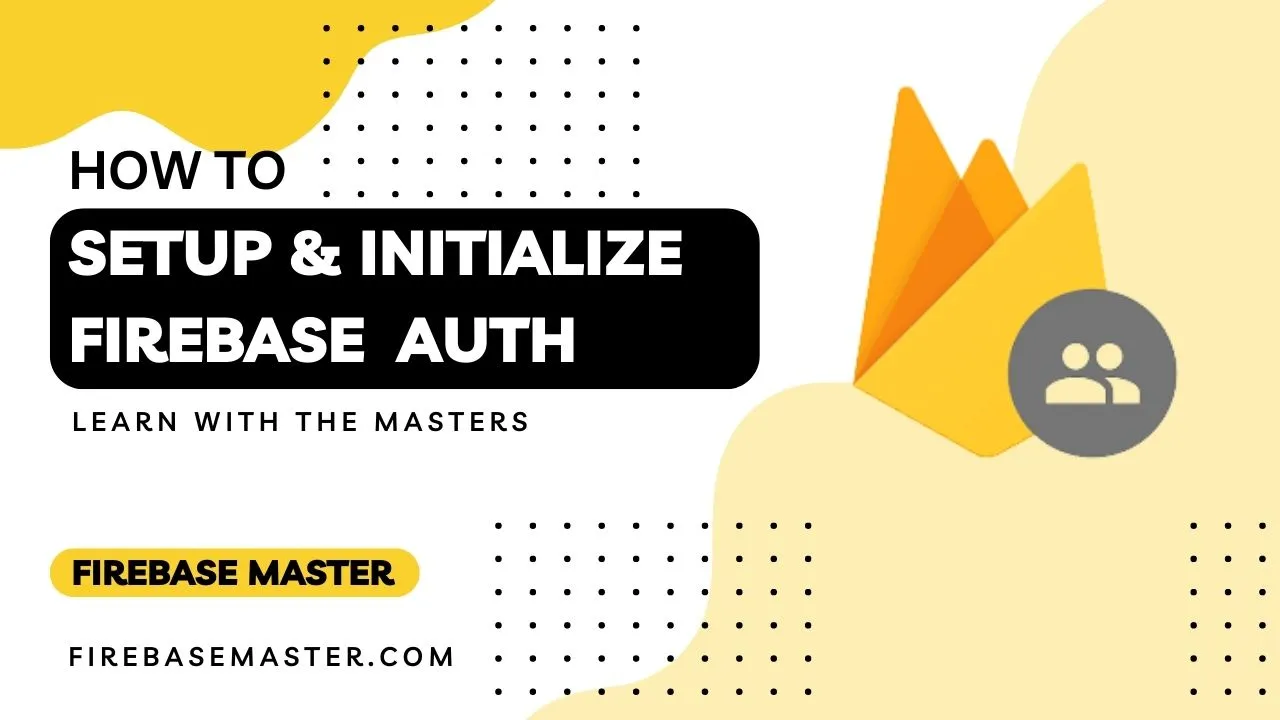
Email/Password
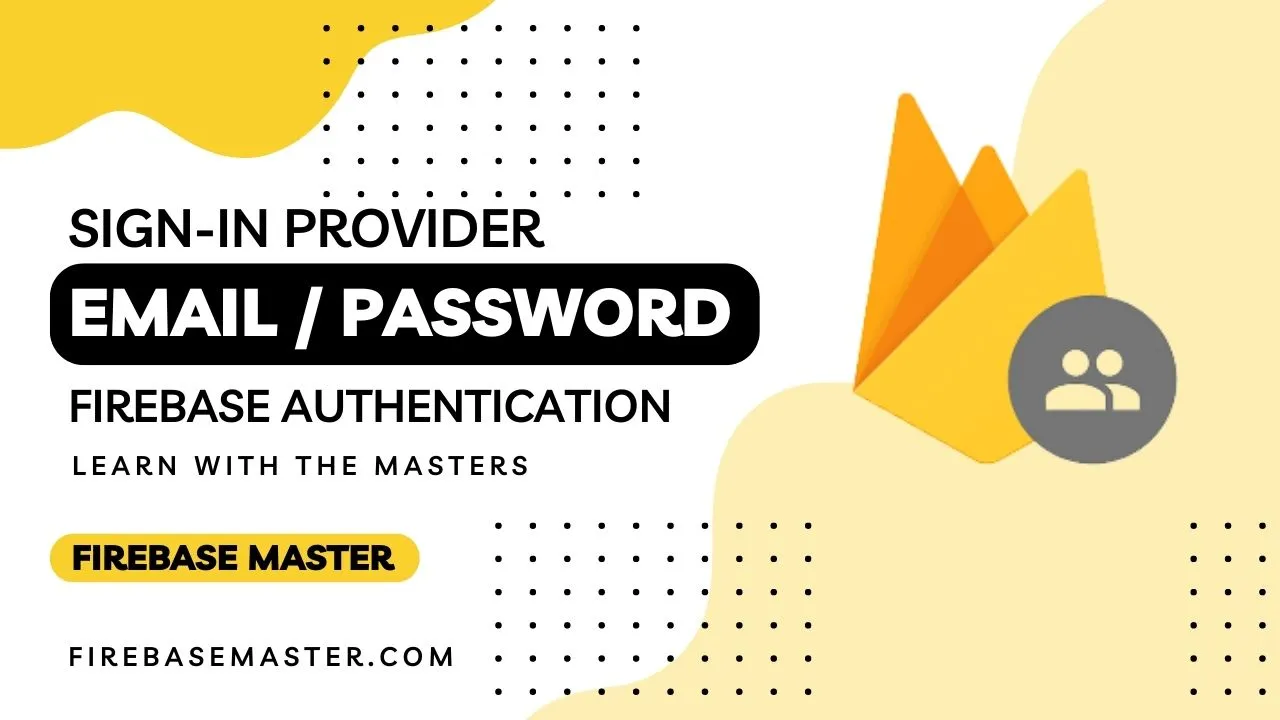
Email link (Passwordless)
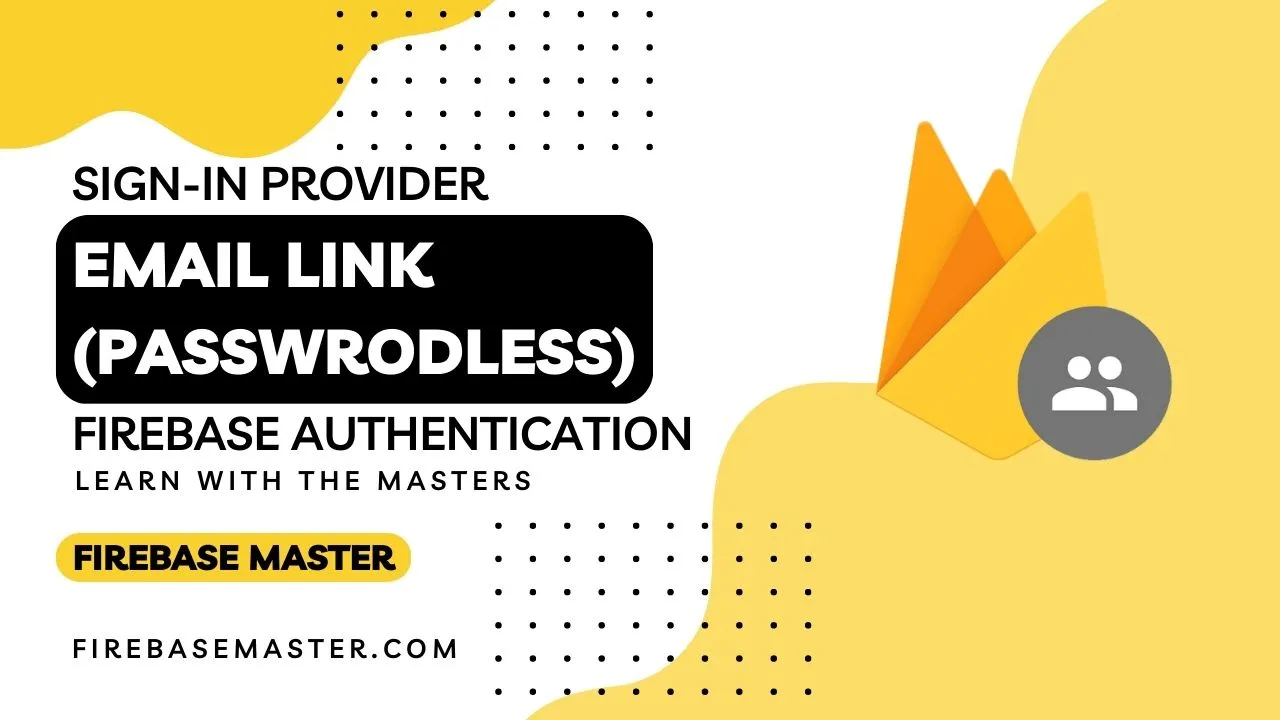
Google OAuth
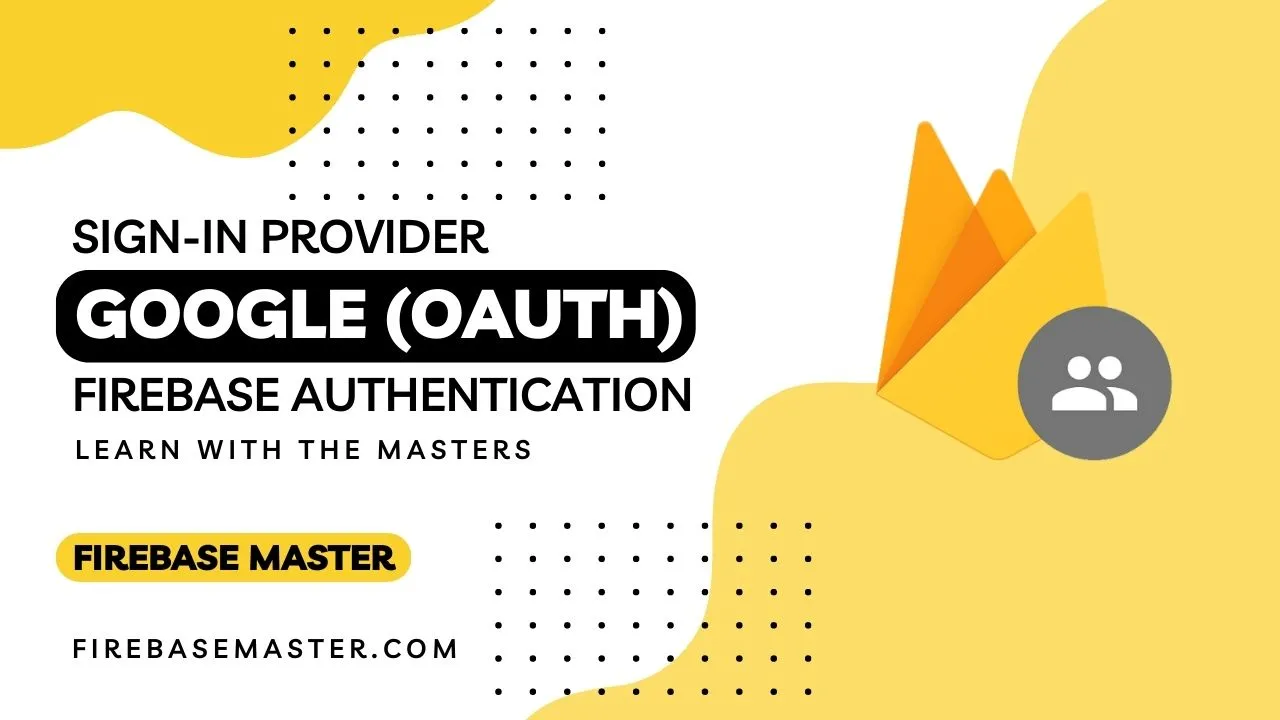
Explore
Copyright © 2024, All rights reserved by Firebase Master